ØxOPOSɆC Crypto Challenges 2019
Write-up for ØxOPOSɆC crypto challenge that involves AES in CTR mode.
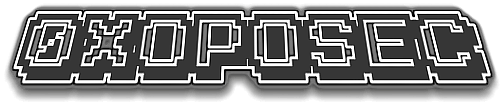
Level 1
This first level is an example of how the known-plaintext attack can be used against the AES-CTR algorithm, if incorrectly implemented.
We're given a ciphered text (C1):
5/KIDoW4XvtTCLZQSQl9GYBmdSC+vj2wDxP4Bbg=
and its plaintext equivalent (P1):
this data is indeed important
And we're asked to decipher a second cipher (C2):
9faAGt62D79tSa1GNhR2FbpgY3mnpyKAEVfrD7E=
We also know that this is an implementation of the AES block cipher in CTR mode, and that both plaintexts were encrypted with the same key and IV.
In these conditions we know that:
C1 XOR C2 = P1 XOR P2
and as such:
P2 = P1 XOR (C1 XOR C2)
To find P2 I created a simple Python script:
Level 2
On this level, we're provided with an example of a valid cipher but not its plaintext equivalent:
22jBDaecKR9Sn4JXzJkxXwY/cAgC9/Gg5JImfEgQLC0=
We also have access to a server which confirms or denies the validity of any given cipher, but it also lets us know when the error is due to invalid padding, which makes it vulnerable to padding oracle attacks.
Instead of creating a "new" Python script, I simply adapted the following PoC: https://github.com/mpgn/Padding-oracle-attack: